Aleš Sýkora / November 28, 2023 / 0 comments
Create category toggle menu with Summary and Details HTML tags
min read / Custom Code, Oxygen Builder, Plugins, WooCommerce, WordPress / Share on: Twitter, LinkedIn, Facebook
Post summary: Today I will show you a great tip for really simple navigation menu. This menu can be used in template which is used for Product Archive and also for product category archives. I build it for one submenu level only. How does it looks? On Shop page Only parent categories are visible. You can open…
Today I will show you a great tip for really simple navigation menu. This menu can be used in template which is used for Product Archive and also for product category archives.
I build it for one submenu level only.
How does it looks?
On Shop page
Only parent categories are visible. You can open the child category list by click on the icon:
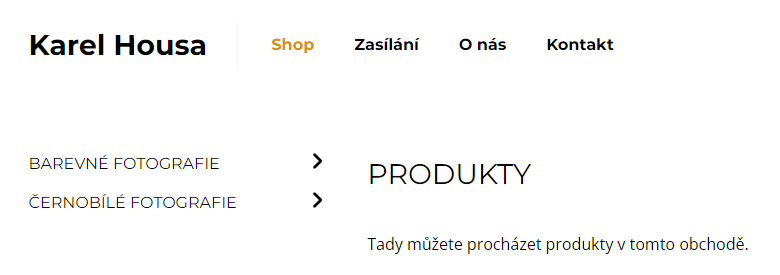
On Parent Category page

On Sub Category page
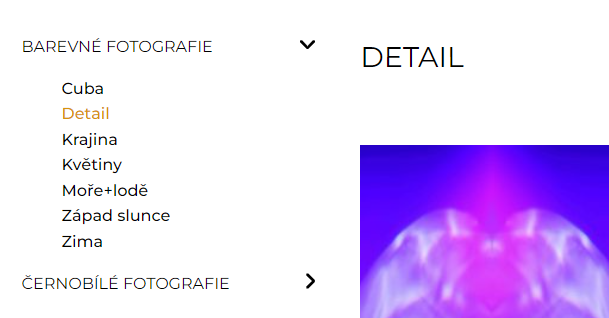
How does it work?
It works with HTML tags <summary> and <details> which are the simplest toggle without .js which you can create. Fast & Furious.
Example:
<details>
<summary>Details</summary>
Something small enough to escape casual notice.
</details>
Details
Something small enough to escape casual notice.
Give me the code, NOW!
Here it is. The code below basically:
- Get list of Product categories
- Check if you are on parent or child category archive
- Add links and <summary> and <details> tags
Code for taxonomy “CATEGORY”
<?php
$parentid = get_queried_object()->parent; //Get ID of current term
if ($parentid == 0) {
$parent = get_queried_object()->term_id;
} else {
$parent = get_queried_object()->parent;
}
$current = get_queried_object()->term_id; //Get ID of current term
$taxonomy = 'category';
$orderby = 'name';
$show_count = 0; // 1 for yes, 0 for no
$pad_counts = 0; // 1 for yes, 0 for no
$hierarchical = 1; // 1 for yes, 0 for no
$title = '';
$empty = 1;
$args = array(
'taxonomy' => $taxonomy,
'orderby' => $orderby,
'show_count' => $show_count,
'pad_counts' => $pad_counts,
'hierarchical' => $hierarchical,
'title_li' => $title,
'hide_empty' => $empty
);
$all_categories = get_categories($args);
foreach ($all_categories as $cat)
{
if ($cat->category_parent == 0)
{
$category_id = $cat->term_id;
if ($parent == $category_id)
{
echo '<details open class="vertical-menu"><summary class="vertical-menu__link"><a href="' . get_term_link($cat->slug, 'category') . '">' . $cat->name . '</a></summary>';
}
else
{
echo '<details class="vertical-menu"><summary class="vertical-menu__link"><a href="' . get_term_link($cat->slug, 'category') . '">' . $cat->name . '</a></summary>';
}
$args2 = array(
'taxonomy' => $taxonomy,
'child_of' => 0,
'parent' => $category_id,
'orderby' => $orderby,
'show_count' => $show_count,
'pad_counts' => $pad_counts,
'hierarchical' => $hierarchical,
'title_li' => $title,
'hide_empty' => $empty
);
$sub_cats = get_categories($args2);
if ($sub_cats)
{
?><ul><?php
foreach ($sub_cats as $sub_category)
{
if ($current == $sub_category->term_id)
{
echo '<li><a class="link--primary" href="' . get_term_link($sub_category->slug, 'category') . '">' . $sub_category->name . '</a></li>';
}
else
{
echo '<li><a href="' . get_term_link($sub_category->slug, 'category') . '">' . $sub_category->name . '</a></li>';
}
}
?></ul></details><?php
}
}
}
?>
Code for WooCommerce product Category:
<?php
$parentid = get_queried_object()->parent; //Get ID of current term
if ($parentid == 0) {
$parent = get_queried_object()->term_id;
} else {
$parent = get_queried_object()->parent;
}
$current = get_queried_object()->term_id; //Get ID of current term
$taxonomy = 'product_cat';
$orderby = 'name';
$show_count = 0; // 1 for yes, 0 for no
$pad_counts = 0; // 1 for yes, 0 for no
$hierarchical = 1; // 1 for yes, 0 for no
$title = '';
$empty = 1;
$args = array(
'taxonomy' => $taxonomy,
'orderby' => $orderby,
'show_count' => $show_count,
'pad_counts' => $pad_counts,
'hierarchical' => $hierarchical,
'title_li' => $title,
'hide_empty' => $empty
);
$all_categories = get_categories($args);
foreach ($all_categories as $cat)
{
if ($cat->category_parent == 0)
{
$category_id = $cat->term_id;
if ($parent == $category_id)
{
echo '<details open class="vertical-menu"><summary class="vertical-menu__link"><a href="' . get_term_link($cat->slug, 'product_cat') . '">' . $cat->name . '</a></summary>';
}
else
{
echo '<details class="vertical-menu"><summary class="vertical-menu__link"><a href="' . get_term_link($cat->slug, 'product_cat') . '">' . $cat->name . '</a></summary>';
}
$args2 = array(
'taxonomy' => $taxonomy,
'child_of' => 0,
'parent' => $category_id,
'orderby' => $orderby,
'show_count' => $show_count,
'pad_counts' => $pad_counts,
'hierarchical' => $hierarchical,
'title_li' => $title,
'hide_empty' => $empty
);
$sub_cats = get_categories($args2);
if ($sub_cats)
{
?><ul><?php
foreach ($sub_cats as $sub_category)
{
if ($current == $sub_category->term_id)
{
echo '<li><a class="link--primary" href="' . get_term_link($sub_category->slug, 'product_cat') . '">' . $sub_category->name . '</a></li>';
}
else
{
echo '<li><a href="' . get_term_link($sub_category->slug, 'product_cat') . '">' . $sub_category->name . '</a></li>';
}
}
?></ul></details><?php
}
}
}
?>
And some CSS… You should edit the classes in the PHP code (especially the link–primary), because I am using Automatic.css.
summary {width:100%; position:relative; list-style: none}
summary::-webkit-details-marker {display: none; }
details summary::after {
content: url("data:image/svg+xml,%3Csvg viewBox='0 0 256 512'%3E%3C!--! Font Awesome Pro 6.1.1 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2022 Fonticons, Inc. --%3E%3Cpath d='M64 448c-8.188 0-16.38-3.125-22.62-9.375c-12.5-12.5-12.5-32.75 0-45.25L178.8 256L41.38 118.6c-12.5-12.5-12.5-32.75 0-45.25s32.75-12.5 45.25 0l160 160c12.5 12.5 12.5 32.75 0 45.25l-160 160C80.38 444.9 72.19 448 64 448z'/%3E%3C/svg%3E");
width: 10px;
position:absolute;
right:0;
}
/* By using [open] we can define different styles when the disclosure widget is open */
details[open] summary::after {
content: url("data:image/svg+xml,%3Csvg viewBox='0 0 384 512'%3E%3C!--! Font Awesome Pro 6.1.1 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2022 Fonticons, Inc. --%3E%3Cpath d='M192 384c-8.188 0-16.38-3.125-22.62-9.375l-160-160c-12.5-12.5-12.5-32.75 0-45.25s32.75-12.5 45.25 0L192 306.8l137.4-137.4c12.5-12.5 32.75-12.5 45.25 0s12.5 32.75 0 45.25l-160 160C208.4 380.9 200.2 384 192 384z'/%3E%3C/svg%3E"); width: 10px;
width: 15px;
position:absolute;
right:0;
}
details ul {list-style: none!important;}
details ul li a {
font-family: 'Montserrat';
font-weight:500;
color:var(--base);
}
Fuel my passion for writing with a beer🍺
Your support not only makes me drunk but also greatly motivates me to continue creating content that helps. Cheers to more discoveries and shared success. 🍻